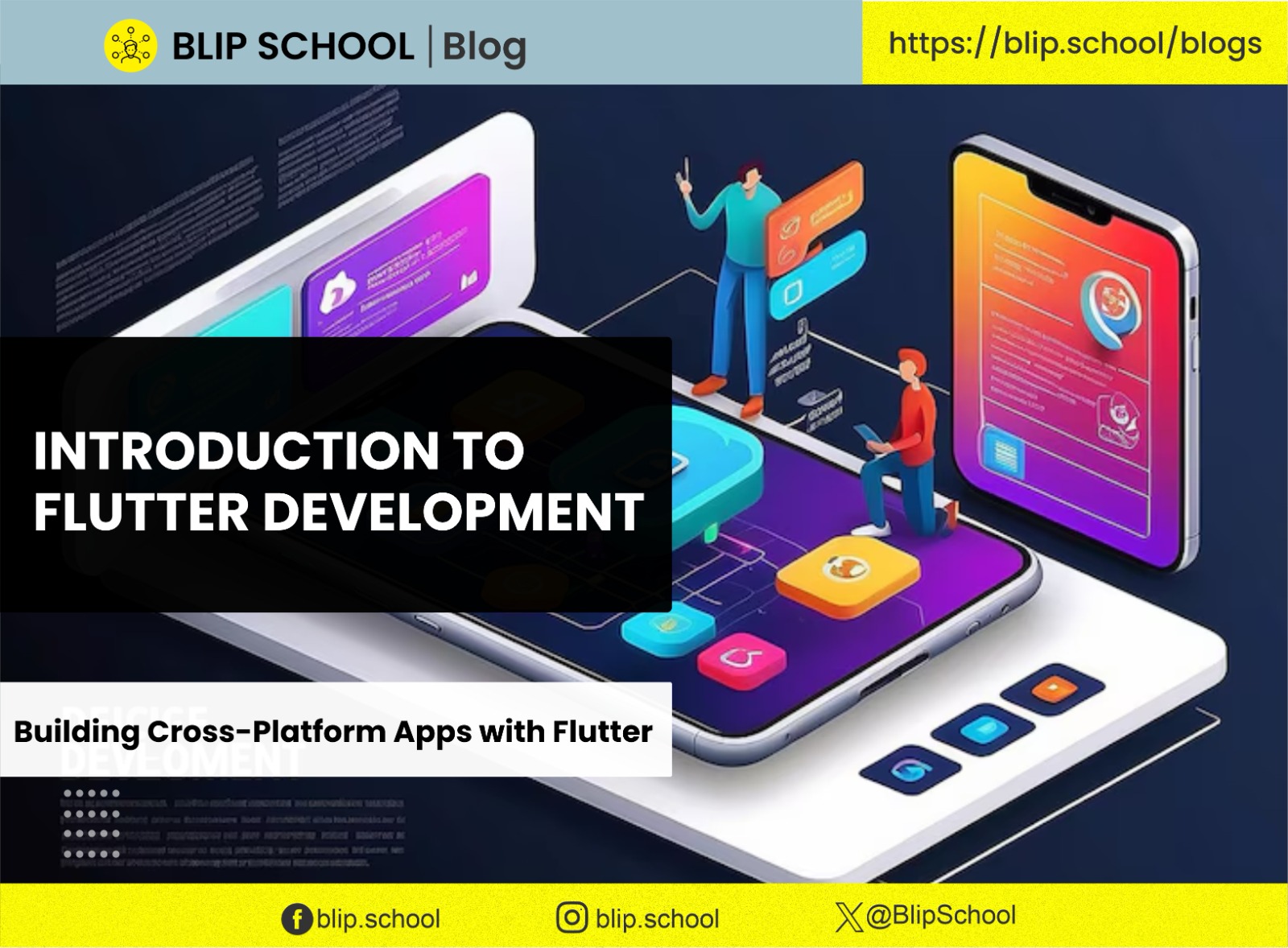
Introduction to Flutter Development: Building Cross-Platform Apps with Flutter
What is Flutter?
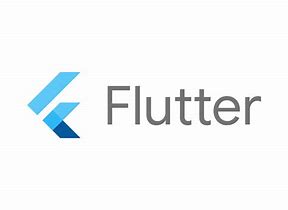
Flutter is an open-source UI software development kit created by Google. It’s used to develop applications for Android, iOS, Linux, Mac, Windows, Google Fuchsia, and the web from a single codebase. This means you can write one app that runs on all major platforms, saving you significant time and effort.
Why Choose Flutter?
There are several reasons why developers choose Flutter for cross-platform app development:
- Single Codebase: Flutter allows developers to write one codebase for two platforms—iOS and Android—saving significant time and effort.
- Hot Reload: This feature enables developers to experiment, build UIs, add features, and fix bugs faster. It allows developers to see the changes in the code immediately in the app, significantly improving the development speed and bringing a huge boost in productivity.
- Customizable Widgets: Flutter provides a rich set of widgets that are customizable, helping developers to create visually appealing apps.
- Native Performance: Despite being a cross-platform framework, Flutter offers close to native performance. Its widgets incorporate all critical platform differences such as scrolling, navigation, icons, and fonts to provide full native performance on both iOS and Android.
The Dart Programming Language
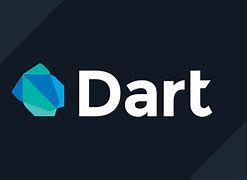
Flutter uses the Dart programming language. Dart is object-oriented and strongly typed. It supports both just-in-time and ahead-of-time compilation, making it ideal for building native performance mobile applications.
Building Cross-Platform Apps with Flutter
Building apps with Flutter involves several key steps:
- Setting up the development environment: This includes installing Flutter and setting up the editor.
- Creating a new project: Once the environment is set up, the next step is to create a new Flutter project.
- Building the UI: Flutter provides a rich set of widgets to create the app’s UI.
- Adding functionality: After the UI is set, the next step is to add functionality to the app.
- Testing and debugging: Flutter offers several ways to test and debug the app.
- Building and deploying: Finally, the app is built and deployed to the app store.
Getting Started with Flutter
To start with Flutter, you first need to install it. Follow the instructions on the official Flutter website to get Flutter up and running on your machine. Once installed, you can verify the installation by running flutter doctor in your terminal/command prompt, which checks your environment and displays a report of the status of your Flutter installation.
Building Your First Flutter App
Once you’ve installed Flutter, you can start building your first Flutter app. Here’s a simple example of a Flutter app:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Welcome to Flutter',
home: Scaffold(
appBar: AppBar(
title: Text('Welcome to Flutter'),
),
body: Center(
child: Text('Hello World'),
),
),
);
}
}
This code creates a new app that displays “Hello World” in the center of the screen. The runApp function takes the given Widget and makes it the root of the widget tree. In this case, our widget tree consists of two widgets, MaterialApp and MyApp.
Flutter’s Ecosystem
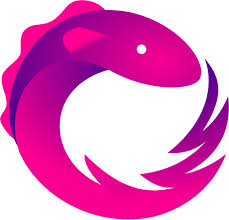
Flutter has a growing ecosystem of packages that makes it easier to quickly implement common features. Some of the popular packages include Dio for networking, RxDart for reactive programming, Provider for state management, and many more.
Learn More with Blip School
If you’re interested in learning more about Flutter development, consider enrolling in our Flutter program at Blip School. Our comprehensive program covers everything from the basics of Flutter to advanced topics, providing you with the skills needed to start building your own cross-platform applications. You’ll get hands-on experience building Flutter apps and learn from experienced developers. We hope to see you there!
Join us at Blip School and start your Flutter journey today!
If you found this article helpful, consider sharing it with others who may benefit from learning about Flutter development. Don't hesitate to leave a comment below with your thoughts, questions, or suggestions for future topics. Subscribe to our newsletter for latest updates on upcoming articles and resources.